Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- NumPy
- 파이썬
- 코딩테스트
- 알고리즘
- programmers
- MySQL
- Matplotlib
- openCV
- Selenium
- 정보처리기사 c언어
- 데이터시각화
- pandas
- javascript
- Algorithm
- 자료구조
- 노마드코딩
- 선그래프
- 프로그래머스
- 알고리즘스터디
- python
- dataframe
- 가상환경
- 백준
- Stack
- Join
- 알고리즘 스터디
- String Method
- queue
- type hint
- aws jupyter notebook
Archives
- Today
- Total
조금씩 꾸준히 완성을 향해
[OpenCV/Python] 이벤트 처리 (키보드, 마우스, 트랙바) 본문
# 키보드 이벤트 처리
▶ 키보드 방향키를 누르면 움직이는 도형의 방향 변경
import numpy as np
import cv2
# 클래스 정의
class move_circle:
def __init__(self, width, height, x, y, R):
self.width = width
self.height = height
self.x = x
self.y = y
self.R = R
self.left, self.right, self.down, self.up = 2, 0, 1, 3
self.direction = self.down
self.key = 0
def key_input(self):
key = cv2.waitKeyEx(1000)
if key == 0x1B:
return 0
elif key == 0x270000: #right
mc.direction = 0
return 1
elif key == 0x280000: #up
mc.direction = 3
return 1
elif key == 0x250000: #left
mc.direction = 2
return 1
elif key == 0x260000: #down
mc.direction = 1
return 1
elif key == 0x2e0000: #del
cv2.destroyAllWindows()
return 0
def direction_change(self):
if self.direction == self.up:
self.x, self.y = (self.x, self.y + 20)
elif self.direction == self.down:
self.x, self.y = (self.x, self.y -20)
elif self.direction == self.right:
self.x, self.y = (self.x + 20, self.y)
elif self.direction == self.left:
self.x, self.y = (self.x - 20, self.y)
def detect_wall(self):
#벽에 부딪혔을 때
if 0 > (self.x - self.R) or self.width < (self.x + self.R):
self.direction = abs(self.direction - 2)
self.direction_change()
elif 0 > (self.y - self.R) or self.height < (self.y + self.R):
self.direction = abs(self.direction - 3) + 1
self.direction_change()
mc = move_circle(512, 512, 256, 256, 50) # 객체 생성
while(True):
if mc.key_input() == 0: # del 키 누르면 종료
break
mc.direction_change()
mc.detect_wall()
img = np.zeros(shape = (mc.width, mc.height, 3), dtype = np.uint8) + 255 # 흰배경
cv2.circle(img, center = (mc.x, mc.y), radius = mc.R,
color = (0, 0, 255), thickness = 4) # 원 그리기
cv2.startWindowThread()
cv2.imshow('Moving', img)
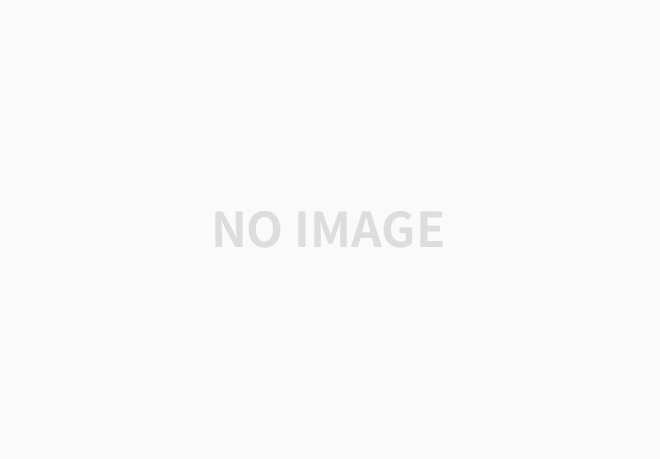
# 마우스 이벤트 처리
- cv2.setMouseCallback(windowName, callback, param=None)
▶ 클릭 이벤트에 따라 도형 생성
def on_mouse(event, x, y, flags, param):
if event == cv2.EVENT_LBUTTONDOWN: # 왼쪽 마우스 클릭
if flags & cv2.EVENT_FLAG_SHIFTKEY: # shift키 + 왼쪽 마우스
cv2.rectangle(param, (x-5, y-5), (x+5, y+5), (255, 0, 0)) # 파란사각형 그리기
else: # 그냥 왼쪽 마우스만 클릭
cv2.circle(param, (x, y), 5, (255, 0, 0), -1) # 빨간 원 그리기
elif event == cv2.EVENT_RBUTTONDOWN: # 오른쪽 마우스 클릭
cv2.circle(param, (x, y), 5, (0, 255, 0), -1) # 초록 원 그리기
cv2.imshow('img', param)
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255 #흰배경 만들기
cv2.imshow('img', img) #흰배경 열기
cv2.setMouseCallback('img', on_mouse, img) #콜백함수 불러오기
cv2.waitKey()
cv2.destroyAllWindows()
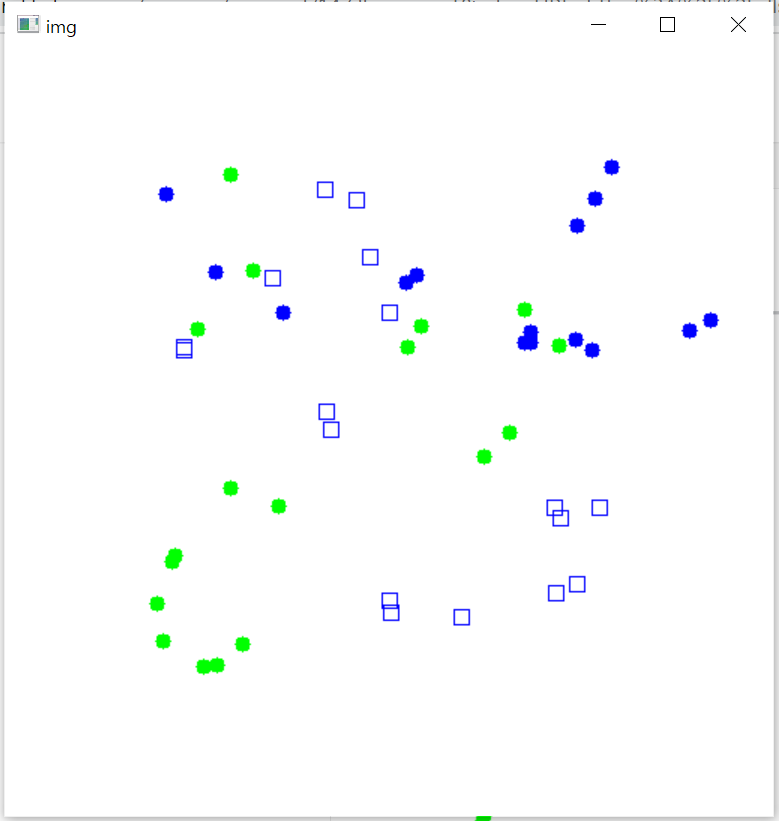
# 트랙바 이벤트 처리
▶ RGB 값 조정 트랙바
def on_change(pos):
global img
r = cv2.getTrackbarPos('R', 'img')
g = cv2.getTrackbarPos('G', 'img')
b = cv2.getTrackbarPos('B', 'img')
img[:] = (b, g, r)
cv2.imshow('img', img)
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8)
cv2.imshow('img', img)
cv2.createTrackbar('R', 'img', 0, 255, on_change)
cv2.createTrackbar('G', 'img', 0, 255, on_change)
cv2.createTrackbar('B', 'img', 0, 255, on_change)
cv2.setTrackbarPos('R', 'img', 0)
cv2.setTrackbarPos('R', 'img', 0)
cv2.setTrackbarPos('R', 'img', 0)
cv2.waitKey()
cv2.destroyAllWindows()
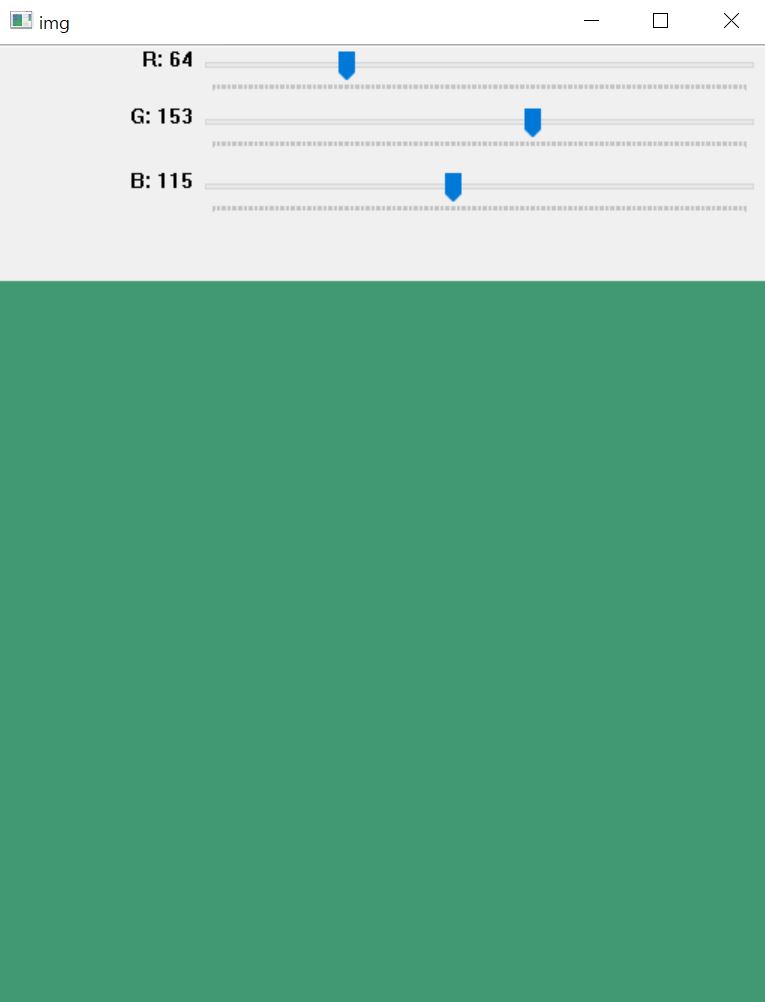
▶ 중첩된 이미지의 알파값(투명도) 조정 트랙바
image1 = './lena.jpg'
image2 = './baboon.jpg'
img1, img2, img3 = 0,0,0
def on_change(pos):
global img3
n = cv2.getTrackbarPos('alpha', 'img')
print('n=', n)
alpha = n/100
print('alpha=', alpha)
img3 = np.uint8(alpha*img2 + (1-alpha)*img1)
cv2.imshow('img', img3)
img1 = cv2.imread(image1)
img2 = cv2.imread(image2)
img3 = img1.copy()
cv2.imshow('img', img3)
cv2.createTrackbar('alpha', 'img', 0, 100, on_change)
cv2.setTrackbarPos('alpha', 'img', 50)
cv2.waitKey()
cv2.destroyAllWindows()
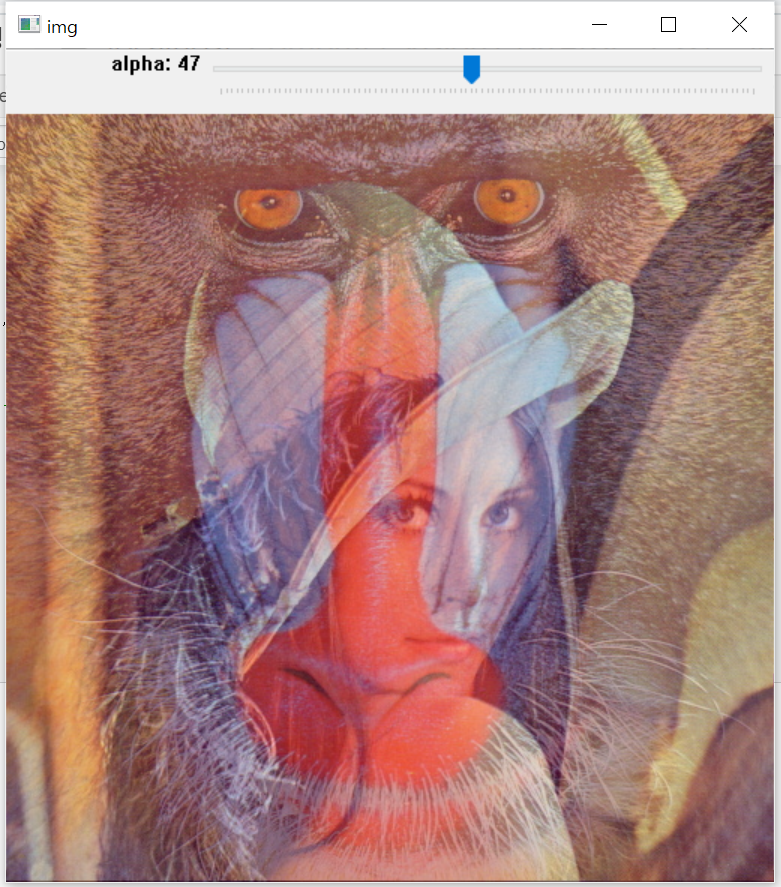