Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 |
Tags
- 정보처리기사 c언어
- Matplotlib
- Algorithm
- 파이썬
- 알고리즘스터디
- queue
- python
- pandas
- 선그래프
- 노마드코딩
- openCV
- aws jupyter notebook
- dataframe
- 가상환경
- Join
- type hint
- javascript
- Selenium
- 백준
- NumPy
- MySQL
- 알고리즘
- 자료구조
- 코딩테스트
- programmers
- 알고리즘 스터디
- String Method
- Stack
- 프로그래머스
- 데이터시각화
Archives
- Today
- Total
조금씩 꾸준히 완성을 향해
[OpenCV/Python] 도형 그리기(line, rectangle, clipLine, circle, ellipse, polylines, ellipse2Poly, fillConvexPoly, fillPoly) 본문
Python/OpenCV
[OpenCV/Python] 도형 그리기(line, rectangle, clipLine, circle, ellipse, polylines, ellipse2Poly, fillConvexPoly, fillPoly)
all_sound 2022. 11. 4. 14:37OpenCV 도형 그리기
import cv2
import numpy as np
# line
cv.line(img, pt1, pt2, color[, thickness[, line_type[, shift[, tipLength]]]]) ->img |
Parameters
img | Image. |
pt1 | The point the arrow starts from. |
pt2 | The point the arrow points to. |
color | Line color. |
thickness | Line thickness. |
line_type | Type of the line. |
shift | Number of fractional bits in the point coordinates. |
tipLength | The length of the arrow tip in relation to the arrow length |
# White 배경 생성 및 pt1-pt2(Red), pt1-pt2(Blue)을 잇는 선 그리기
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255 # 흰 배경이미지 생성
# 꼭지점 지정
pt1 = 0, 0
pt2 = 500, 0
pt3 = 0, 500
# blue_color = (255, 0, 0)
# green_color = (0, 255, 0)
# red_color = (0, 0, 255)
# white_color = (255, 255, 255)
cv2.line(img, pt1, pt2, (0, 0, 255), 5)
cv2.line(img, pt2, pt3, (255, 0, 0), 5)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
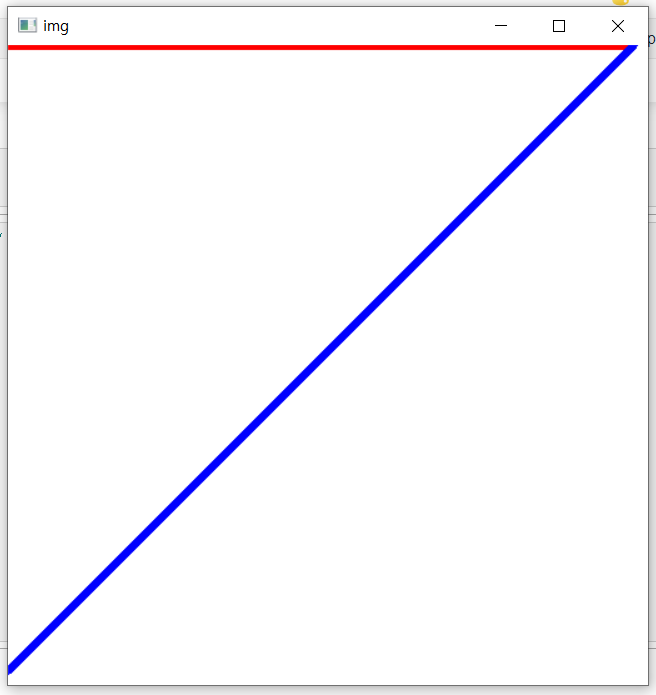
# rectangle
cv.rectangle(img, pt1, pt2, color[, thickness[, lineType[, shift]]]) -> img |
cv.rectangle(img, rec, color[, thickness[, lineType[, shift]]]) -> img |
Parameters
img | Image. |
pt1 | Vertex of the rectangle. |
pt2 | Vertex of the rectangle opposite to pt1 . |
color | Rectangle color or brightness (grayscale image). |
thickness | Thickness of lines that make up the rectangle. Negative values, like FILLED, mean that the function has to draw a filled rectangle. |
lineType | Type of the line. |
shift | Number of fractional bits in the point coordinates. |
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
pt1 = 0, 0
pt2 = 400, 400
cv2.rectangle(img, pt1, pt2, (0, 255, 0), 5)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
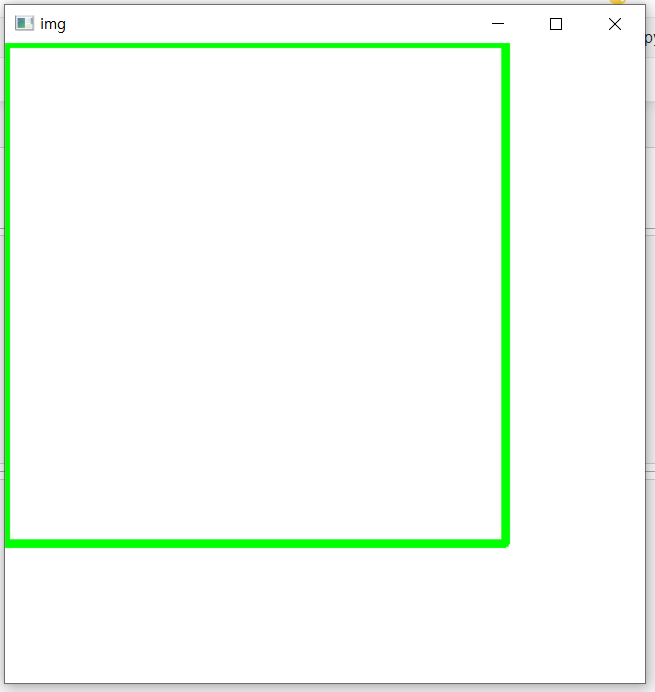
# line + rectangle
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
pt1 = 0, 0
pt2 = 500, 0
pt3 = 0, 500
pt4 = 30, 30
pt5 = 400, 400
cv2.line(img, pt1, pt2, (0, 0, 255), 5)
cv2.line(img, pt1, pt3, (255, 0, 0), 5)
cv2.rectangle(img, pt4, pt5, (0, 255, 0), 3)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
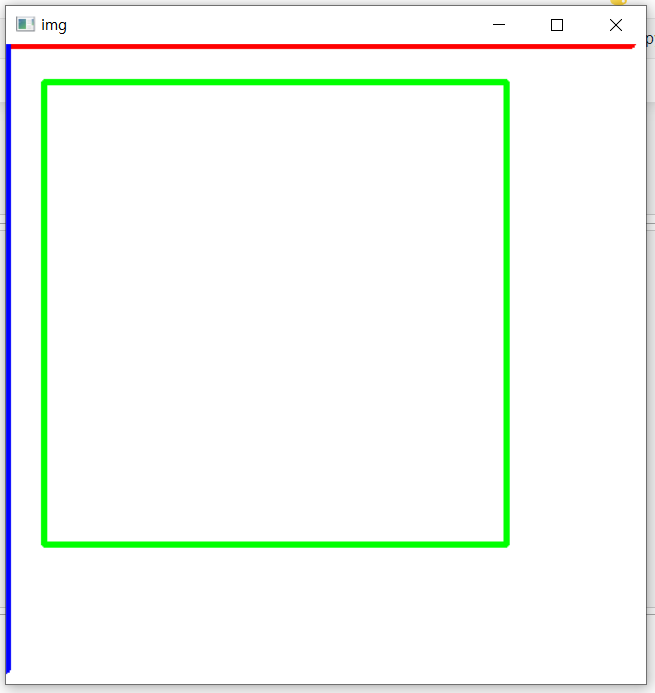
# clipLine
- 직사각형과 직선이 만나는 교차점을 구하는 함수
cv.clipLine(imgRect, pt1, pt2) ->retval, pt1, pt2 |
Parameters
imgSize | Image size. The image rectangle is Rect(0, 0, imgSize.width, imgSize.height) . |
pt1 | First line point. |
pt2 | Second line point. |
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255 #흰배경 설정
x1, x2 = 100, 400
y1, y2 = 100, 400
cv2.rectangle(img, (x1, y1), (x2, y2), (0, 255, 0), 3) #사각형 그리기
pt1 = 120, 50
pt2 = 300, 500
cv2.line(img, pt1, pt2, (255, 0, 0), 3) #직선 그리기
imgRect = (x1, y1, x2-x1, y2-y1)
retval, rpt1, rpt2 = cv2.clipLine(imgRect, pt1, pt2) # 사각형과 직선의 교점구하기
print('retval:', retval)
print('rpt1:', rpt1)
print('rp2:', rpt2)
if retval: # 접점 그리기
cv2.circle(img, rpt1, radius= 8, color = (0, 0, 255),thickness=-1)
cv2.circle(img, rpt2, radius=8, color=(0, 0, 255), thickness=-1)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
#retval: True
#rpt1: (140, 100)
#rp2: (260, 399)
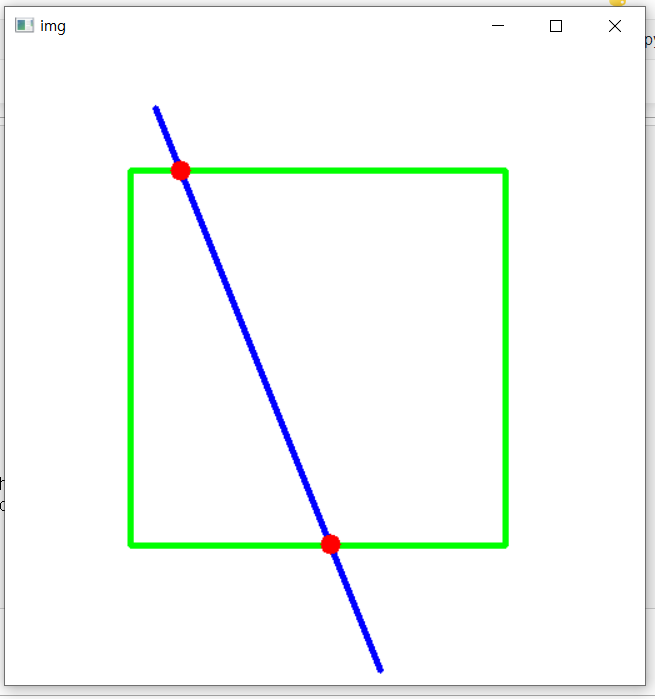
# circle
cv.circle(img, center, radius, color[, thickness[, lineType[, shift]]]) ->img |
Parameters
img | Image where the circle is drawn. |
center | Center of the circle. |
radius | Radius of the circle. |
color | Circle color. |
thickness | Thickness of the circle outline, if positive. Negative values, like FILLED, mean that a filled circle is to be drawn. |
lineType | Type of the circle boundary. |
shift | Number of fractional bits in the coordinates of the center and in the radius value. |
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255 #흰배경 설정
cx = img.shape[0]//2 #배경의 중앙점 설정
cy = img.shape[1]//2
#서로 다른 지름의 원 그리기
cv2.circle(img, (cx, cy), radius=200, color=(0, 0, 255), thickness=2)
cv2.circle(img, (cx, cy), radius=100, color=(0, 255, 0), thickness=2)
cv2.circle(img, (cx, cy), radius=20, color=(255, 0, 0), thickness=2)
cv2.circle(img, (cx, cy), radius=1, color=(0, 80, 200), thickness=2)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
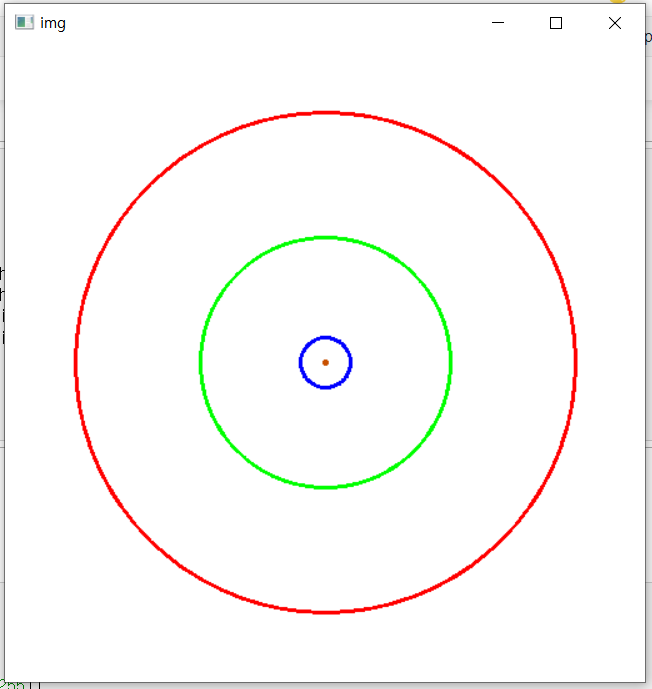
# ellipse
- 타원을 그리는 함수
cv.ellipse(img, center, axes, angle, startAngle, endAngle, color[, thickness[, lineType[, shift]]]) -> img |
cv.ellipse(img, box, color[, thickness[, lineType]]) -> img |
Parameters
img | Image. |
center | Center of the ellipse. |
axes | Half of the size of the ellipse main axes. |
angle | Ellipse rotation angle in degrees. |
startAngle | Starting angle of the elliptic arc in degrees. |
endAngle | Ending angle of the elliptic arc in degrees. |
color | Ellipse color. |
thickness | Thickness of the ellipse arc outline, if positive. Otherwise, this indicates that a filled ellipse sector is to be drawn. |
lineType | Type of the ellipse boundary. |
shift | Number of fractional bits in the coordinates of the center and values of axes. |
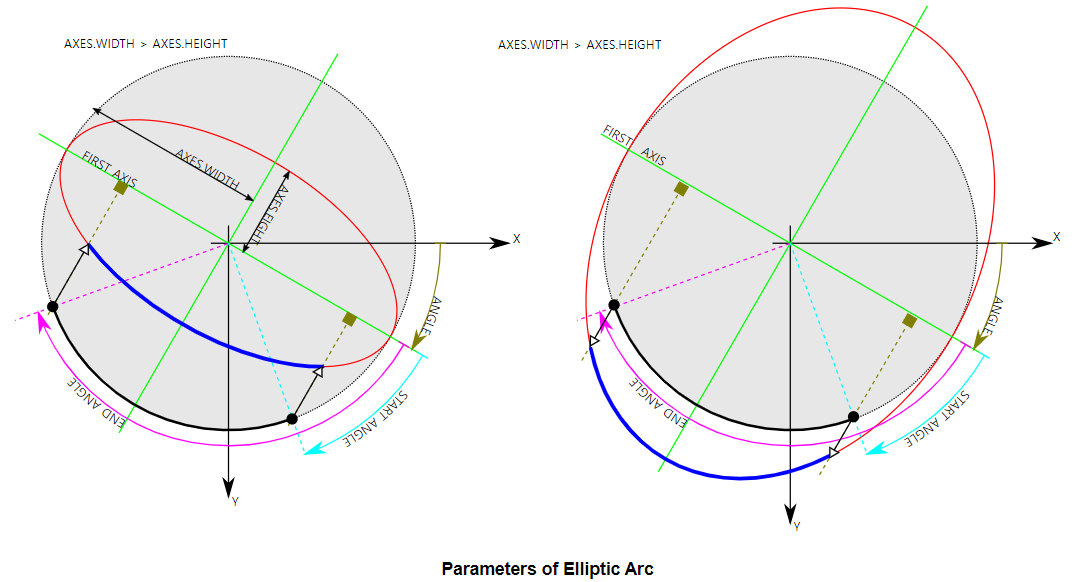
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
ptCenter = (img.shape[0] //2, img.shape[1] //2)
size = (200, 100)
cv2.ellipse(img, ptCenter, size, 0, 0, 360, color=(0, 0, 255))
cv2.ellipse(img, ptCenter, size, 45, 0, 360, color=(0, 255, 0), thickness=1)
cv2.ellipse(img, ptCenter, size, 45, 90, 150, color=(0, 40, 50), thickness=3)
cv2.ellipse(img, ptCenter, size, 20, 0, 360, color=(255, 0, 0), thickness=5)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
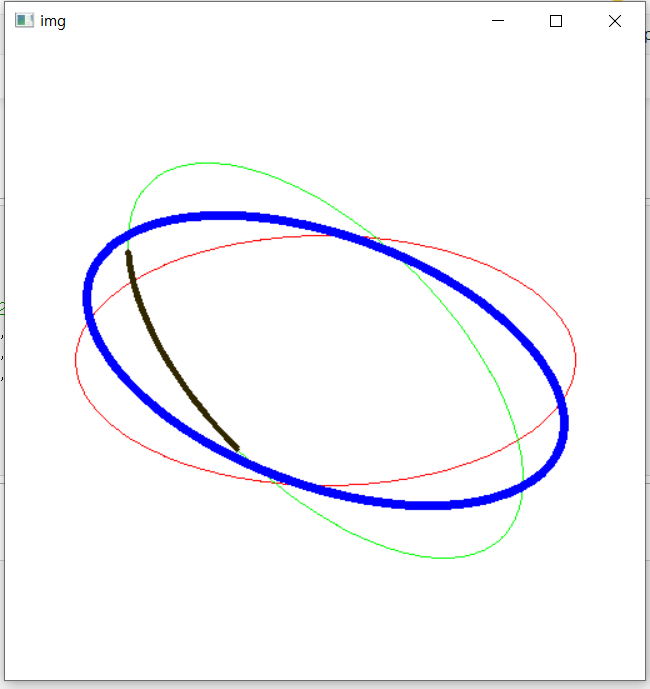
- box 옵션 지정
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
ptCenter = (img.shape[0] //2, img.shape[1] //2)
size = (200, 100)
box = (ptCenter,size,0)
cv2.ellipse(img, box, (0, 0, 255), 3)
box = (ptCenter,size,45)
cv2.ellipse(img, box, (0, 255, 0), 3)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
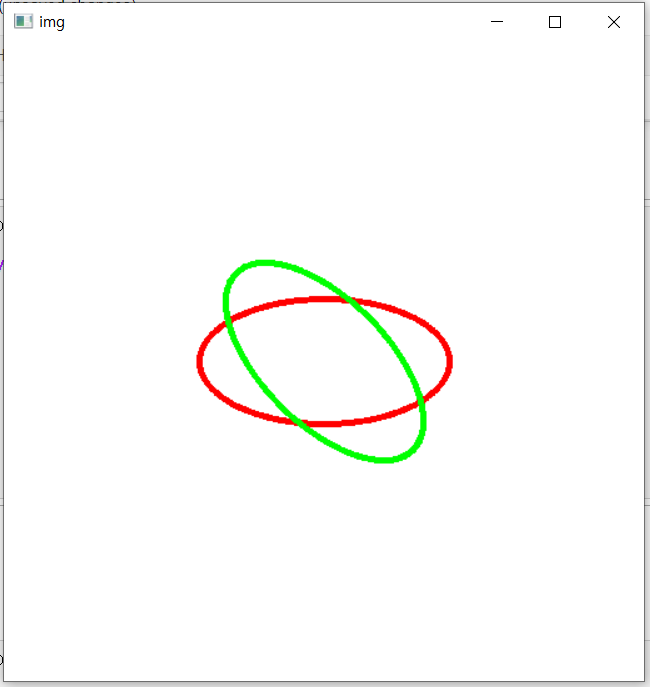
# polylines
- 다각형 그리는 함수
cv.polylines(img, pts, isClosed, color[, thickness[, lineType[, shift]]]) ->img |
Parameters
img | Image. |
pts | Array of polygonal curves. |
isClosed | Flag indicating whether the drawn polylines are closed or not. If they are closed, the function draws a line from the last vertex of each curve to its first vertex. |
color | Polyline color. |
thickness | Thickness of the polyline edges. |
lineType | Type of the line segments. |
shift | Number of fractional bits in the vertex coordinates. |
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
pts1 = np.array([[100, 100], [200, 100],[200, 200], [100, 200]])
pts2 = np.array([[400, 100], [300, 200],[400,200]])
cv2.polylines(img, [pts1, pts2], isClosed=True, color=(0, 0, 255), thickness = 2)
pts3 = np.array([[100, 300], [200, 300],[200,400],[100,400]])
cv2.polylines(img, [pts3], isClosed=False, color=(0, 255, 0), thickness = 2)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
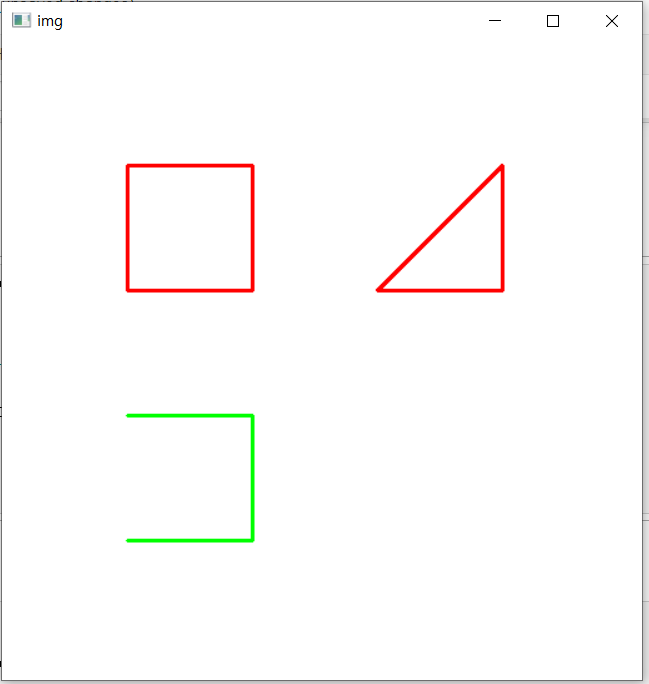
# 도형 혼합
- ellipse + polylines
cv.ellipse2Poly(center, axes, angle, arcStart, arcEnd, delta) ->pts |
Parameters
center | Center of the arc. |
axes | Half of the size of the ellipse main axes. |
angle | Rotation angle of the ellipse in degrees. |
arcStart | Starting angle of the elliptic arc in degrees. |
arcEnd | Ending angle of the elliptic arc in degrees. |
delta | Angle between the subsequent polyline vertices. It defines the approximation accuracy. |
pts | Output vector of polyline vertices. |
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
ptCenter = (img.shape[0]//2, img.shape[1]//2)
size = (200, 100)
cv2.ellipse(img, ptCenter, size, 0, 0, 360, color=(0, 0, 255))
pts1 = cv2.ellipse2Poly(ptCenter, size, 0, 0, 360, delta=50)
cv2.polylines(img, [pts1], isClosed=True, color=(255, 0, 0))
cv2.ellipse(img, ptCenter, size, 45, 0, 360, color=(0, 255, 0))
pts2 = cv2.ellipse2Poly(ptCenter, size, 45, 0, 360, delta=50)
cv2.polylines(img, [pts2], isClosed=True, color=(255, 0, 0))
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
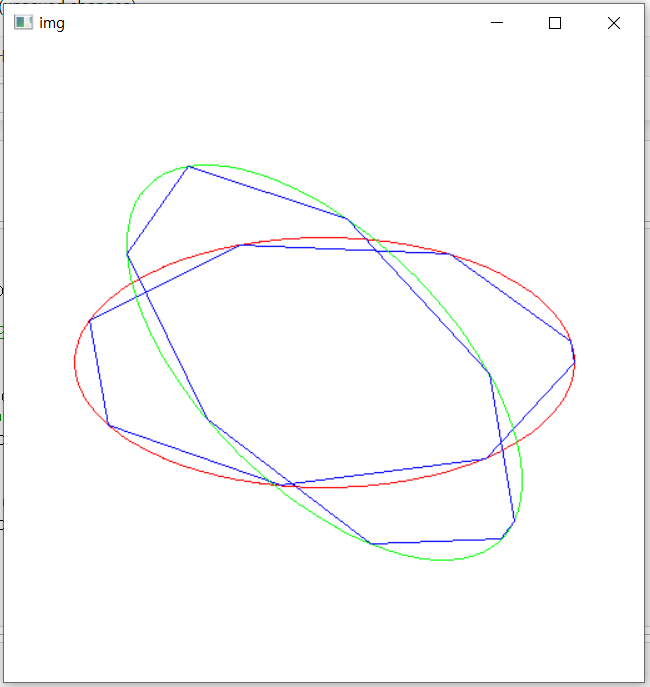
- rotated rectangle
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
ptCenter, size = (img.shape[0]//2, img.shape[1]//2), 200
for angle in range(0, 90, 5):
rect = (ptCenter, (size, size), angle)
box = cv2.boxPoints(rect).astype(np.int32)
r = np.random.randint(256)
g = np.random.randint(256)
b = np.random.randint(256)
cv2.polylines(img, [box], True, (r, g, b), 2)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
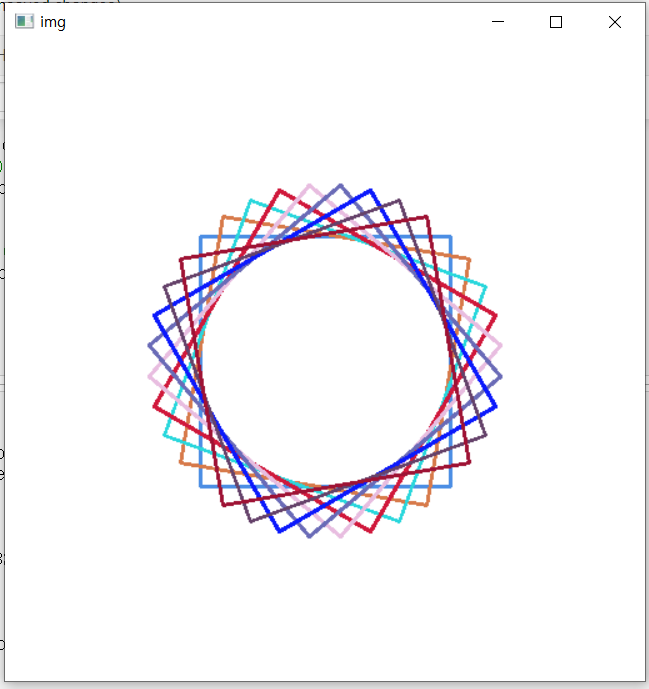
# fillConvexPoly / fillPoly
- fillConvexPoly : 채워진 블록 다각형을 그리는 함수
cv.fillConvexPoly(img, points, color[, lineType[, shift]]) ->img |
Parameters
img | Image. |
points | Polygon vertices. |
color | Polygon color. |
lineType | Type of the polygon boundaries. |
shift | Number of fractional bits in the vertex coordinates. |
- fillPoly : 채워진 다각형을 그리는 함수
cv.fillPoly(img, pts, color[, lineType[, shift[, offset]]]) ->img |
Parameters
img | Image. |
pts | Array of polygons where each polygon is represented as an array of points. |
color | Polygon color. |
lineType | Type of the polygon boundaries. |
shift | Number of fractional bits in the vertex coordinates. |
offset | Optional offset of all points of the contours. |
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
pts1 = np.array([[100, 100], [200, 100], [200, 200], [100, 200]])
pts2 = np.array([[400, 100], [300, 200], [400,200]])
cv2.fillConvexPoly(img, pts1, (0, 0, 255))
cv2.fillPoly(img, [pts2], (255, 0, 0))
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
img1 = np.zeros((400, 400, 3), np.uint8)
img2 = np.zeros((400, 400, 3), np.uint8)
pt1 = np.array([[100, 100], [270, 110], [300, 330], [170, 170], [150,250]],np.int32)
cv2.fillConvexPoly(img1, pt1, (0, 255, 0))
cv2.fillPoly(img2, [pt1], (255, 0, 0))
cv2.imshow('convex', img1)
cv2.imshow('poly', img2)
cv2.waitKey()
cv2.destroyAllWindows()
'Python > OpenCV' 카테고리의 다른 글
[OpenCV/Python] 이벤트 처리 (키보드, 마우스, 트랙바) (0) | 2022.11.13 |
---|---|
[OpenCV/Python] 히스토그램(Histogram) 그래프 & 스트레칭 & 평활화 (normalize, equalize) (0) | 2022.11.13 |
[OpenCV/Python] 임계값(Threshold) 처리 (BINARY, BINARY_INV, TRIANGLE, OTSU 등) (0) | 2022.11.12 |
[OpenCV/Python] 영상접근법(화소접근, 컬러접근, ROI, 복사, 컬러 채널 분리&병합, 색변환, 기하변환, 기본연산) (0) | 2022.11.12 |
[OpenCV/Python] 이미지 불러오기 & 읽기 & 저장하기 (0) | 2022.11.04 |